Equipment Expansion Pack
I'm writing new weapons for the game, I'm mostly filling gaps and adding more types of weapons like flamethrowers, grenade launchers, rocket launchers, living weapons; using new types of damage like corrosive, freezing, psionic or poison.
For the moment I'm focusing on ranged weapons, then I'll move to melee weapons, then accessories, armors and drones, with consumables as a stretch goal.
============================================================================
Spreadsheet
This is a spreadsheet which is a bit more beautiful and easier to understand.
Nice spreadsheet, click here.
-------------------------------------------------------------------------------------------------------------------------------------
Weapon descriptions
I've compiled the weapon descriptions in an independent doc, so you don't have to rummage through the code looking for them.
Weapon descriptions, click here.
-------------------------------------------------------------------------------------------------------------------------------------
Weapon design
In the following document I keep my design philosophy, some keywords and concepts as well as basic info.
Design philosophy, click here.
-------------------------------------------------------------------------------------------------------------------------------------
Raw code
Raw code might be a bit confusing; at the end of this post you can find a tutorial on how to read it.
Raw code, click here.
============================================================================
I also need your comments, suggestions and help with proofreading.
Did I miss a t or an s? did I write can when I meant can't? did I write something in mutant English? weird grammar? is a word missing? the usual.
Ideas and requests for new weapons are also welcome.
Do you want something specific? Simply ask, if it's not to overly complicated I'll probably write it or if you have the basic idea with numbers, a name and a description, I'll code it.
============================================================================
Reading and understanding the code:
Note: Just in case; if for whatever reason I leave this project unfinished, anyone who wants to finish the job has my permission, no need to ask, no credit needed, just go ahead.
Update 28/07/2017: Ranged weapons are now fully planned and I'll begin coding and writing them soon. I've also made a full, ranged weapon rebalance to make ranged weapons more consistent and standardized. there is now a better sense of progression, but there are still plenty of choices. Check the spreadsheet for more info.
Update 02/08/2017: Descriptions for all ranged weapons are already done. Proofreading and coding are next. Feel free to check the descriptions here and point out any mistakes, weird grammar, etc.
Update 12/08/2017: Coding is done, testing comes next.
Update: 16/08/2017: Testing is done. Final edit phase.
I'm writing new weapons for the game, I'm mostly filling gaps and adding more types of weapons like flamethrowers, grenade launchers, rocket launchers, living weapons; using new types of damage like corrosive, freezing, psionic or poison.
For the moment I'm focusing on ranged weapons, then I'll move to melee weapons, then accessories, armors and drones, with consumables as a stretch goal.
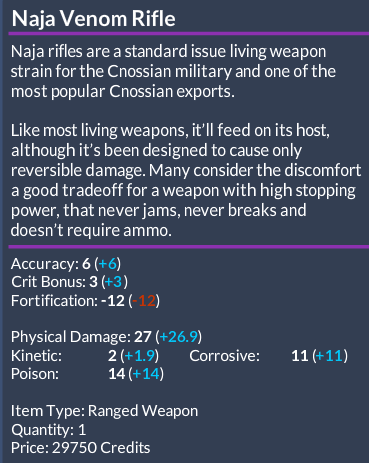
============================================================================
Spreadsheet
This is a spreadsheet which is a bit more beautiful and easier to understand.
Nice spreadsheet, click here.
-------------------------------------------------------------------------------------------------------------------------------------
Weapon descriptions
I've compiled the weapon descriptions in an independent doc, so you don't have to rummage through the code looking for them.
Weapon descriptions, click here.
-------------------------------------------------------------------------------------------------------------------------------------
Weapon design
In the following document I keep my design philosophy, some keywords and concepts as well as basic info.
Design philosophy, click here.
-------------------------------------------------------------------------------------------------------------------------------------
Raw code
Raw code might be a bit confusing; at the end of this post you can find a tutorial on how to read it.
Raw code, click here.
============================================================================
I also need your comments, suggestions and help with proofreading.
Did I miss a t or an s? did I write can when I meant can't? did I write something in mutant English? weird grammar? is a word missing? the usual.
Ideas and requests for new weapons are also welcome.
Do you want something specific? Simply ask, if it's not to overly complicated I'll probably write it or if you have the basic idea with numbers, a name and a description, I'll code it.
============================================================================
Reading and understanding the code:
package classes.Items.Guns //This classifies the item and indicates where to store it.
import classes.Engine.Combat.DamageTypes.DamageFlag; //Imports are other scripts your weapon will depend on, in this case this indicates a damage flag, which is a flag that indicates how to modify the damage against certain armor flags. This is an optional import, necessary if you have a damage flag, for example bullet or energy weapon.
import classes.GameData.CombatAttacks; //This indicates the weapon uses an special attack modifier that's stored in the CombatAttacks script. Drones and certain weapons rely on this for more complex types of attacks. This is an optional import.
import classes.Engine.Combat.DamageTypes.TypeCollection;
import classes.GLOBAL;
import classes.GameData.TooltipManager;
import classes.ItemSlotClass;
import classes.StringUtil; //all those imports are necessary for a weapon to function.
public class FreezeRay extends ItemSlotClass
public function FreezeRay() //these 2 lines indicate the name of your weapon to the code, so other pieces of code can call for the weapon.
super();
this._latestVersion = 1; //don't touch this
this.quantity = 1;
this.stackSize = 1; //this indicated the quantity and stack size of the item, you shouldn't touch the quantity. 1 is the standard for gear, consumables and other items usually go up to 10.
this.type = GLOBAL.RANGED_WEAPON; //the type of item, in this case a ranged weapon.
this.shortName = "Freeze R."; //the short name you'll see in your inventory
this.longName = "LM22 “Freeze Ray” kinetic decelerator"; //the long name you'll see when the item is equipped an in the description.
TooltipManager.addFullName(this.shortName,StringUtil.toTitleCase(this.longName)); //this function shows the full name of the weapon in game. Don't touch this.
this.description = "a freeze ray"; //basic description
this.tooltip = "The LM22 is an experimental weapon; an upgraded version of its predecessor the LM19. Like the LM19, it also operates by slowing down the movement of atomic and subatomic particles. As an experimental weapon, its reliability is a bit lower than that of the finished product."; //long description
this.attackVerb = "shoot"; //this indicates the attack verb, certain scenes might call this function.
this.attackNoun = "energy beam"; //the attack noun. Certain scenes might call for this function for example: you [attackverb] with your [weapon], its [attacknoun] hits the target.
TooltipManager.addTooltip(this.shortName,this.tooltip); //this function adds the description in-game.
this.basePrice = 27500; //base price in credits
baseDamage.freezing.damageValue = 25; //how much damage the weapon does and its type, in this case 25 points of freezing damage.
baseDamage.addFlag(DamageFlag.ENERGY_WEAPON); //a damage flag
this.addFlag(GLOBAL.ITEM_FLAG_ENERGY_WEAPON); //a global flag, I'm not entirely sure but I think this is just an informative and decorative flag, it does nothing per se, but tags the weapon for other stuff.
this.attack = 2; //accuracy value
this.defense = 0; //defense value
this.shieldDefense = 0; //shield defense value
this.shields = 0; //total shield value
this.sexiness = 0; //sexiness value, note: cannot go under -10
this.critBonus = 2; //critical hit value
this.evasion = 0; //evasion value
this.fortification = 0; //max HP modifier
this.version = _latestVersion; //don't touch this
attackImplementor = CombatAttacks.FZRAttackImpl; //this is a modified attack, in this case this one applies a debuff. Some weapons might have it, they are coded independently.
resistances.kinetic.resistanceValue = 10; //damage resistance value, in this case 10% kinetic damage resistance. Weapons don't usually have this, I just added it here as an example.
Damage types:
HP damage:: Kinetic, Electric, Burning, Freezing, Corrosive, Poison, True/Unresistable Damage.
Lust damage: Psionic, Drug, Pheromone, Tease, True/Unresistable Lust.
Damage flags:
FlagNames[PENETRATING] = "Penetrating";
FlagNames[ABLATIVE] = "Ablative";
FlagNames[PLATED] = "Plated";
FlagNames[CRUSHING] = "Crushing";
FlagNames[BULLET] = "Bullet";
FlagNames[LASER] = "Laser";
FlagNames[MIRRORED] = "Mirrored";
FlagNames[CRYSTAL] = "Crystal";
FlagNames[PSIONIC] = "Psionic";
FlagNames[NULLIFYING] = "Nullifying";
FlagNames[AMPLIFYING] = "Amplifying";
FlagNames[EXPLOSIVE] = "Explosive";
FlagNames[ENERGY_WEAPON] = "Energy Weaponry";
FlagNames[GROUNDED] = "Grounded";
FlagNames[BYPASS_SHIELD] = "Shield Bypass";
FlagNames[ONLY_SHIELD] = "Targets Shield";
FlagNames[EASY] = "Easy";
FlagNames[CHANCE_APPLY_BURN] = "Burn DoT Chance";
FlagNames[DRAINING] = "Draining";
FlagNames[GREATER_DRAINING] = "Greater Draining";
FlagNames[VAMPIRIC] = "Vampiric";
FlagNames[GREATER_VAMPIRIC] = "Greater Vampiric";
FlagNames[CRYSTALGOOARMOR] = "Crystal Goo Armor";
FlagNames[SYDIANARMOR] = "Sydian Armor";
FlagNames[CHANCE_APPLY_STUN] = "Stun Chance";
Armor flags:
Weapon Flags: Indicate what type of attack your weapon uses, this flags are used as tags to calculate resistances.
Goo and sydian armor: those are NPC modifiers that influence their armor resistances.
import classes.Engine.Combat.DamageTypes.DamageFlag; //Imports are other scripts your weapon will depend on, in this case this indicates a damage flag, which is a flag that indicates how to modify the damage against certain armor flags. This is an optional import, necessary if you have a damage flag, for example bullet or energy weapon.
import classes.GameData.CombatAttacks; //This indicates the weapon uses an special attack modifier that's stored in the CombatAttacks script. Drones and certain weapons rely on this for more complex types of attacks. This is an optional import.
import classes.Engine.Combat.DamageTypes.TypeCollection;
import classes.GLOBAL;
import classes.GameData.TooltipManager;
import classes.ItemSlotClass;
import classes.StringUtil; //all those imports are necessary for a weapon to function.
public class FreezeRay extends ItemSlotClass
public function FreezeRay() //these 2 lines indicate the name of your weapon to the code, so other pieces of code can call for the weapon.
super();
this._latestVersion = 1; //don't touch this
this.quantity = 1;
this.stackSize = 1; //this indicated the quantity and stack size of the item, you shouldn't touch the quantity. 1 is the standard for gear, consumables and other items usually go up to 10.
this.type = GLOBAL.RANGED_WEAPON; //the type of item, in this case a ranged weapon.
this.shortName = "Freeze R."; //the short name you'll see in your inventory
this.longName = "LM22 “Freeze Ray” kinetic decelerator"; //the long name you'll see when the item is equipped an in the description.
TooltipManager.addFullName(this.shortName,StringUtil.toTitleCase(this.longName)); //this function shows the full name of the weapon in game. Don't touch this.
this.description = "a freeze ray"; //basic description
this.tooltip = "The LM22 is an experimental weapon; an upgraded version of its predecessor the LM19. Like the LM19, it also operates by slowing down the movement of atomic and subatomic particles. As an experimental weapon, its reliability is a bit lower than that of the finished product."; //long description
this.attackVerb = "shoot"; //this indicates the attack verb, certain scenes might call this function.
this.attackNoun = "energy beam"; //the attack noun. Certain scenes might call for this function for example: you [attackverb] with your [weapon], its [attacknoun] hits the target.
TooltipManager.addTooltip(this.shortName,this.tooltip); //this function adds the description in-game.
this.basePrice = 27500; //base price in credits
baseDamage.freezing.damageValue = 25; //how much damage the weapon does and its type, in this case 25 points of freezing damage.
baseDamage.addFlag(DamageFlag.ENERGY_WEAPON); //a damage flag
this.addFlag(GLOBAL.ITEM_FLAG_ENERGY_WEAPON); //a global flag, I'm not entirely sure but I think this is just an informative and decorative flag, it does nothing per se, but tags the weapon for other stuff.
this.attack = 2; //accuracy value
this.defense = 0; //defense value
this.shieldDefense = 0; //shield defense value
this.shields = 0; //total shield value
this.sexiness = 0; //sexiness value, note: cannot go under -10
this.critBonus = 2; //critical hit value
this.evasion = 0; //evasion value
this.fortification = 0; //max HP modifier
this.version = _latestVersion; //don't touch this
attackImplementor = CombatAttacks.FZRAttackImpl; //this is a modified attack, in this case this one applies a debuff. Some weapons might have it, they are coded independently.
resistances.kinetic.resistanceValue = 10; //damage resistance value, in this case 10% kinetic damage resistance. Weapons don't usually have this, I just added it here as an example.
Damage types:
HP damage:: Kinetic, Electric, Burning, Freezing, Corrosive, Poison, True/Unresistable Damage.
Lust damage: Psionic, Drug, Pheromone, Tease, True/Unresistable Lust.
Damage flags:
FlagNames[PENETRATING] = "Penetrating";
FlagNames[ABLATIVE] = "Ablative";
FlagNames[PLATED] = "Plated";
FlagNames[CRUSHING] = "Crushing";
FlagNames[BULLET] = "Bullet";
FlagNames[LASER] = "Laser";
FlagNames[MIRRORED] = "Mirrored";
FlagNames[CRYSTAL] = "Crystal";
FlagNames[PSIONIC] = "Psionic";
FlagNames[NULLIFYING] = "Nullifying";
FlagNames[AMPLIFYING] = "Amplifying";
FlagNames[EXPLOSIVE] = "Explosive";
FlagNames[ENERGY_WEAPON] = "Energy Weaponry";
FlagNames[GROUNDED] = "Grounded";
FlagNames[BYPASS_SHIELD] = "Shield Bypass";
FlagNames[ONLY_SHIELD] = "Targets Shield";
FlagNames[EASY] = "Easy";
FlagNames[CHANCE_APPLY_BURN] = "Burn DoT Chance";
FlagNames[DRAINING] = "Draining";
FlagNames[GREATER_DRAINING] = "Greater Draining";
FlagNames[VAMPIRIC] = "Vampiric";
FlagNames[GREATER_VAMPIRIC] = "Greater Vampiric";
FlagNames[CRYSTALGOOARMOR] = "Crystal Goo Armor";
FlagNames[SYDIANARMOR] = "Sydian Armor";
FlagNames[CHANCE_APPLY_STUN] = "Stun Chance";
Armor flags:
- Ablative: 25% resistant to Penetrating, 15% resistant to Bullet, 25% vulnerable to Crushing.
- Amplifying: 25% vulnerable to Psionic, +25% outgoing psionic damage.
- Crystal: 40% resistant to Laser, 40% vulnerable to Explosive.
- Mirrored: 90% resistant to Laser.
- Nullifying: 25% resistant to Psionic, -25% outgoing psionic damage.
- Plated: 25% resistant to Crushing, 15% resistant to Bullet, 25% vulnerable to Penetrating.
- Easy: easy mode flag, +50% to all resistances.
Weapon Flags: Indicate what type of attack your weapon uses, this flags are used as tags to calculate resistances.
- Penetrating
- Crushing
- Bullet
- Laser
- Psionic
- Explosive
- Energy Weapon
- Shield Bypass (ignores shields)
- Target Shields (only deals shield damage)
- Apply Burn Chance (can deal extra DoT burning damage)
- Stun Chance (might stun the target)
- Draining (steals 50% of shield damage dealt)
- Greater Draining (steals 90% of shield damage dealt)
- Vampiric (steals 50% of HP damage dealt)
- Greater Vampiric (steals 90% of HP damage dealt)
Goo and sydian armor: those are NPC modifiers that influence their armor resistances.
Note: Just in case; if for whatever reason I leave this project unfinished, anyone who wants to finish the job has my permission, no need to ask, no credit needed, just go ahead.
Update 28/07/2017: Ranged weapons are now fully planned and I'll begin coding and writing them soon. I've also made a full, ranged weapon rebalance to make ranged weapons more consistent and standardized. there is now a better sense of progression, but there are still plenty of choices. Check the spreadsheet for more info.
Update 02/08/2017: Descriptions for all ranged weapons are already done. Proofreading and coding are next. Feel free to check the descriptions here and point out any mistakes, weird grammar, etc.
Update 12/08/2017: Coding is done, testing comes next.
Update: 16/08/2017: Testing is done. Final edit phase.
Last edited: